Google Pay is currently available with our latest JavaScript SDK.
How to Set Up Google Pay
In order to accept payments via Google Pay, you will need to enable it in the Merchant Portal. To do so:
1. Log into your SandBox or Production merchant portal
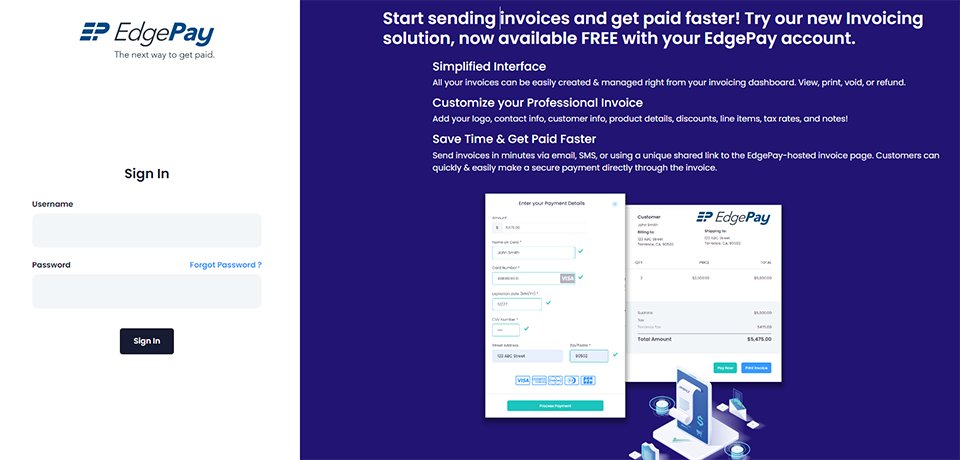
2. Click on Settings on the left menu dropdown
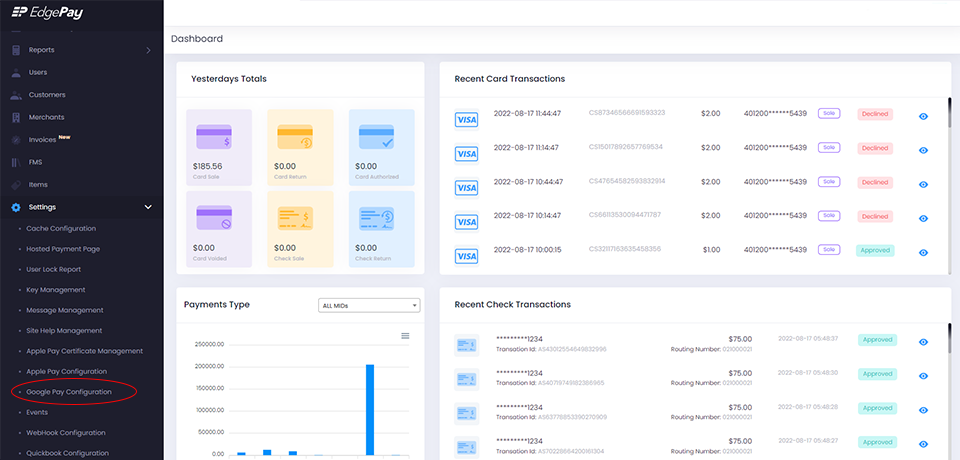
3. Click on the Google Pay Configuration link
4. Click on the Enable button
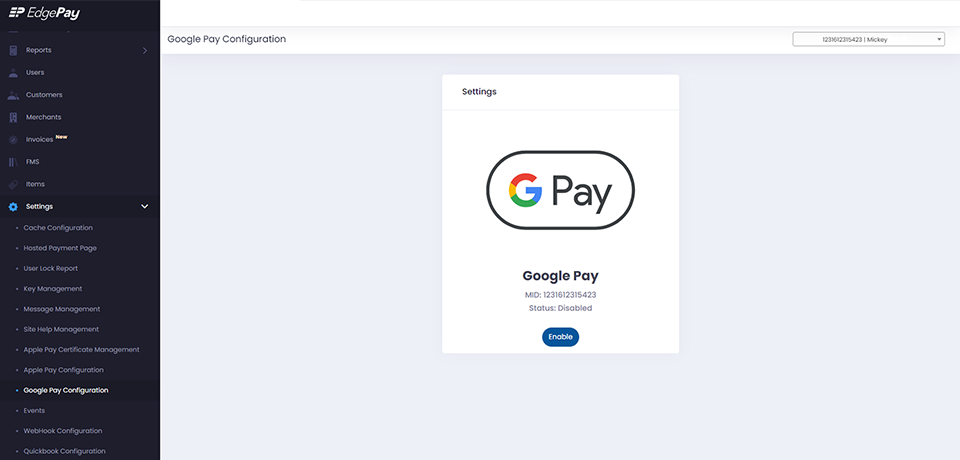
In Production, you will also need to work with Google to go live.
The following links will help you be ready for accepting Google Pay payments in production:
Overview:
Google Pay Web developer documentation
Integration Checklist:
Google Pay Web integration checklist
Brand Guidelines:
Google Pay Web Brand Guidelines
Production Access
Request production access to the Google Pay API via the Business Console
JavaScript SDK
You will need to include the following script tags to load both Google Pay and EdgePay’s JS libraries:
SandBox
<script
type="text/javascript" src="https://js.edgepayportal.com/2.0.0/js/sandbox/client.min.js"></script>
Production
<script
type="text/javascript" src="https://js.edgepayportal.com/2.0.0/js/prod/client.min.js"></script>
Google Pay API JavaScript Library:
<script
async src="https://pay.google.com/gp/p/js/pay.js" onload="googlePayOnload()"></script>
Initialization
Initialize the EdgePay JS client and Google Pay client:
EdgePay.init({ authKey: 'Your Auth Key' }); //You should get this key from your backend
var googlePayOnLoad = async function () {
paymentsClient = new google.payments.api.PaymentsClient({ environment: 'TEST' });
}
Check and add the Google Pay button if the browser supports Google Pay:
isReadyToPayRequestResponse = await EdgePay.googlePay.getIsReadyToPayRequest();
var isReadyToPayRequest;
if (isReadyToPayRequestResponse.success) {
isReadyToPayRequest = isReadyToPayRequestResponse.isReadyToPayRequest;
paymentsClient.isReadyToPay(isReadyToPayRequest)
.then(function (response) {
if (response.result) {
// Add the Google Pay payment button
// Define the "processGooglePayPayment()" function to be triggered when
// a customer clicks on the Google Pay button
const button = paymentsClient.createButton({ onClick: () => processGooglePayPayment() });
document.getElementById('container').appendChild(button);
}
})
.catch(function (err) {
console.error(err);
});
}
else {
console.log("IsReadyToPayRequest error: ", isReadyToPayRequestResponse.errorResponse);
}
IsReadyToPayRequest Object:The IsReadyToPayRequest object is generated automatically by the EdgePay SDK so you don't have to set its values manually.
These are the values generated:
- allowedAuthMethods: Both methods by default (PAN_ONLY and CRYPTOGRAM_3DS)
- allowedCardNetworks: All networks enabled in the Merchant portal for your merchant
- gateway: Our gateway name "globalelectronictechnology"
- gatewayMerchantId: Your merchant ID
{ "type": "CARD",
"parameters": {
"allowedAuthMethods": ["PAN_ONLY", "CRYPTOGRAM_3DS"],
"allowedCardNetworks": ["AMEX", "DISCOVER", "JCB", "MASTERCARD", "VISA"]
}
}
Requesting a Payment
Request a Google Pay token when the Google Pay button is clicked. Once the token is received from Google, pass it to the EdgePay getToken method to get a Pivot token back that you can send to your backend to submit the payment.
var processGooglePayPayment = function () {
var paymentDataRequest = isReadyToPayRequest;
//Example on how to modify the paymentDataRequest object
//Define the transaction information
paymentDataRequest.transactionInfo = {
"totalPriceStatus": "FINAL",
"totalPrice": 1.00,
"currencyCode": "USD"
};
//Provide a user-visible merchant name and your Google Merchant ID
paymentDataRequest.merchantInfo = {
merchantName: 'Example Merchant',
merchantId: '12345678901234567890'
};
//We require all Google Pay transactions to include the billing postal code
//Below you can see an example of how to modify the paymentDataRequest object
//to request the billing information
var cardPaymentMethod = paymentDataRequest.allowedPaymentMethods[0];
cardPaymentMethod.parameters.billingAddressRequired = true;
cardPaymentMethod.parameters.billingAddressParameters = {
format: 'MIN'
};
paymentsClient.loadPaymentData(paymentDataRequest).then(function (paymentData) {
//After receiving the Google Pay payment data, pass it to the getToken
//method to receive an EdgePay Pivot token
EdgePay.googlePay.getToken({
paymentData,
onSuccess: function (response) {
console.log("success ", response);
//Submit the token (response.successResponse.tokenID)
//and payment information (amount, zip code, etc) to your backend for processing.
},
onError: function (response) {
console.log("error", response);
}
})
}).catch(function (err) {
console.error(err);
});
}